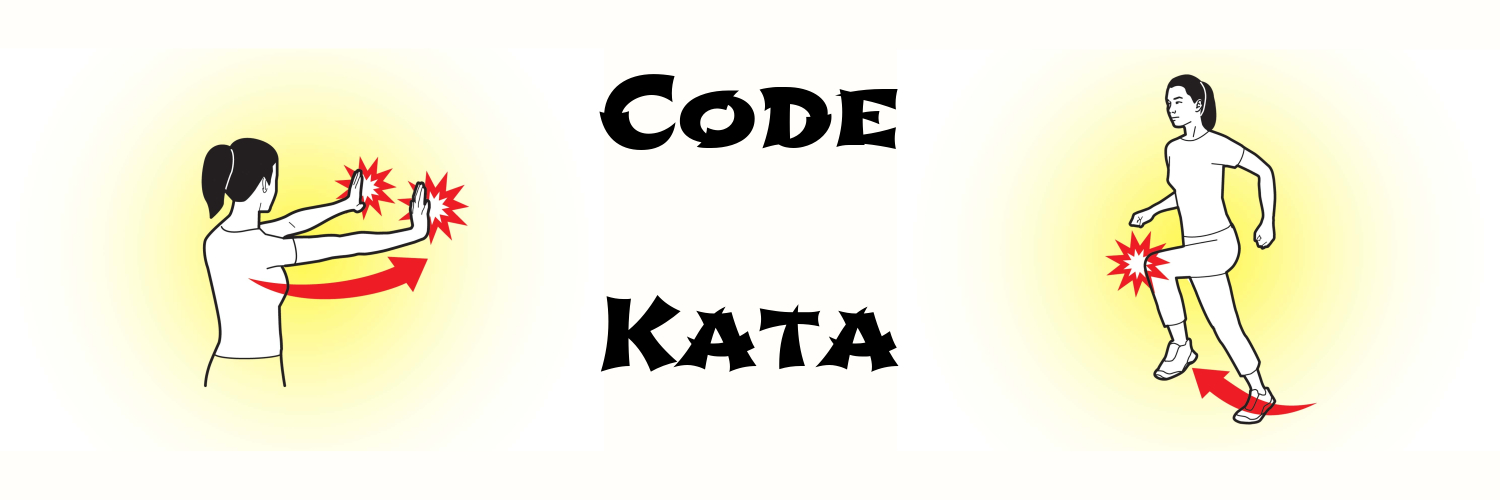
Problem
Suppose a binary tree structure looks like this:
{:val value :L <left-node> :R <right-node>}
Write a function to balance insert node into the tree.
Solution
(defn insert [tree x]
(cond (nil? tree)
{:val x :L nil :R nil}
(< x (:val tree))
(assoc tree :L (insert (:L tree) x))
:else
(assoc tree :R (insert (:R tree) x))))
Note
Here is the function to traverse the tree:
(defn traverse [tree]
(when tree
(concat (traverse (:L tree)) [(:val tree)] (traverse (:R tree)))))
Here is a helper function to create a tree.
(defn create-tree [& xs]
(reduce (fn [result next] (xconj result next)) nil xs))
Share this post
Twitter
Google+
Facebook
Reddit
LinkedIn
StumbleUpon
Email